How to Build a Telegram Bot for Google Calendar Integration


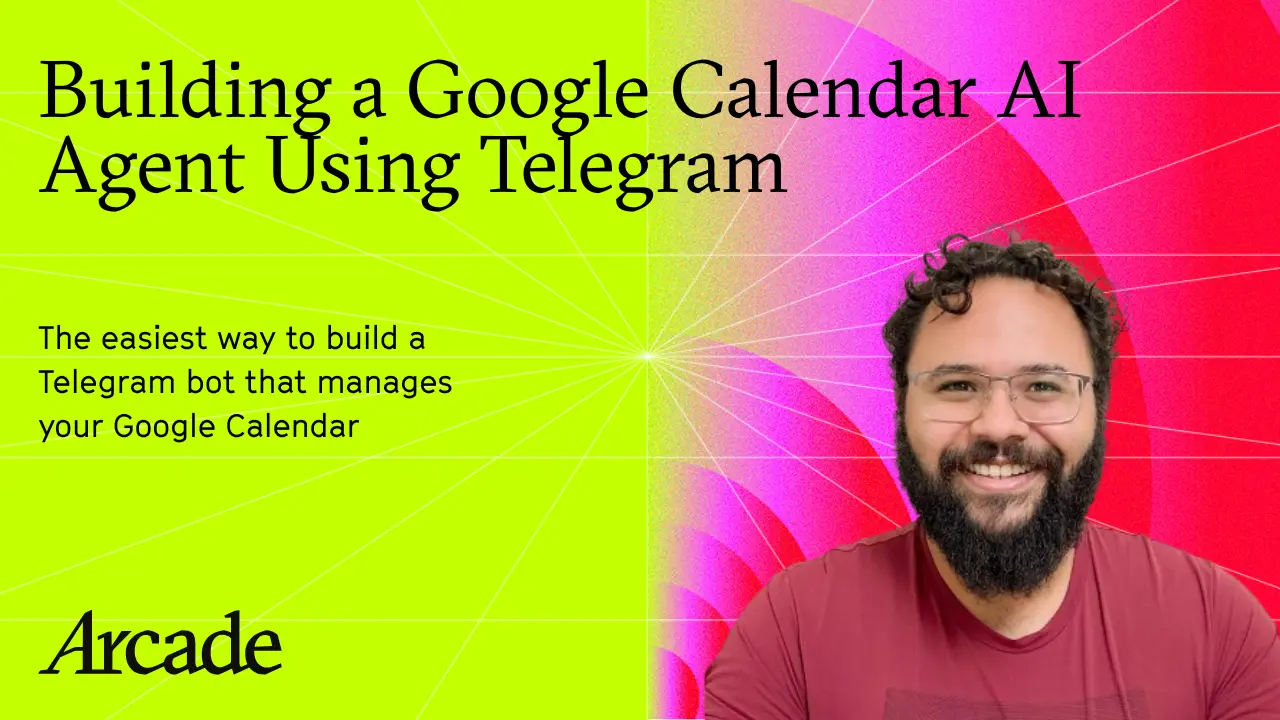
What You'll Learn
In this tutorial, you'll build a Telegram bot that uses natural language to create and manage Google Calendar events. We'll show you how to:
- Set up a Telegram bot that understands calendar commands
- Connect securely to Google Calendar using Arcade.dev tools
- Parse natural language into calendar events using LLMs
- Delegate the entire authentication flow to Arcade.dev
Time to complete: 30 minutes
Prerequisites: Basic JavaScript knowledge, Telegram account, Google account
The Problem with Calendar Bots
Building bots that interact with Google Calendar typically requires juggling:
- Complex OAuth 2.0 authentication flows
- Google API credential management
- Token storage and refresh logic
- An Express server for handling OAuth redirects
This complexity is why many developers stay stuck into a single platform or abandon calendar integration projects before they reach production. It’s the same kind of complexity that divides native apps from being published for both iPhones and Android phones. For mobile developers, a solution is found in tools like React-native or Flutter, which enable cross-platform apps that share the same code underneath.
The API-based world has a similar problem. Once I’ve integrated all the pieces into WhatsApp or Telegram, adding the next platform requires reinventing the wheel to fit the platform. Maintaining that app means fixing the same bugs in multiple, often incompatible, code bases. Add the complexity of integrating intelligent LLMs into the mix, and you can see why not many are willing to ship to more than a single platform.
Arcade.dev allows us to remove this complexity entirely. We can literally delegate the complexity of running not only multiple traditional API calls to the Arcade Engine, but we also get an interface to Agentic building blocks, letting us seamlessly integrate the latest models into our products.
The Arcade.dev Solution
We'll refactor an existing Telegram calendar bot to use Arcade.dev, which:
- Handles the entire OAuth flow automatically
- Provides ready-made toolkit functions for calendar operations
- Reduces tons lines of authentication code to a single function call
- Eliminates the need for a separate Express.JS server
Removing complexity
Replace Google OAuth Arcade Authentication
Before:
import express, { Request, Response } from 'express';
import { google } from 'googleapis';
import { OAuth2Client } from 'google-auth-library';
// ... setup Express.JS app with callbacks for auth,
// handle expired tokens and refreshes,
// manual API calls, 80 lines at least
After:
// no express js app
// no OAuth2Client
// no raw API clients
const { Arcade } = require('@arcade-dev/sdk');
// Auth is now handled with
// a single function call
const authResponse = await arcade.auth.start(
user_id,
"google",
{scopes:[/* set scopes */],}
);
Simplify Calendar Operations with direct tool calling
Replace direct Google API calls with Arcade toolkit functions. These are originally designed for LLMs to use, but we can call them directly with way better DX compared to the Google API client.
// List calendars using Arcade
const toolResponse = await arcade.tools.execute({
tool_name: "Google.ListCalendars",
input: {show_deleted: true,show_hidden: true,},
user_id: user_id,
});
// Create an event
const toolResponse = await arcade.tools.execute({
tool_name: "Google.CreateEvent",
input: {
calendar_id: calendarId,
summary: eventData.summary,
description: eventData.description,
start_datetime: eventData.start_time,
end_datetime: eventData.end_time,
},
user_id: user_id,
});
Testing Your Bot
- Clone the repo
- Start your bot with npm start
- Open Telegram and find your bot
- Send the /auth command and complete Google authentication
- Try creating an event with natural language: "Meeting with Alex tomorrow at 3pm for 1 hour to discuss the project"
Conclusion
You've now built a Telegram bot that can:
- Securely authenticate with Google Calendar
- List and manage multiple calendars
- Create events using natural language
- Provide a conversational interface for calendar management
By using Arcade.dev, we eliminated the complexity of OAuth flows and API integration, focusing instead on the bot's functionality and user experience.
Next Steps
- Add reminder functionality
- Implement event editing capabilities
- Create recurring events
- Build multi-user calendar sharing
FAQs
Q: Do I need to handle token refresh with Arcade.dev?
A: No, Arcade.dev manages token lifecycle automatically.
Q: Can this approach work with Microsoft Calendar or other providers?
A: Yes, Arcade.dev supports multiple calendar providers with the same pattern.
Q: How secure is the authentication process?
A: Arcade.dev uses industry-standard OAuth 2.0 without storing user credentials. It follows the best security practices of least-privilege and requires the least amount of auth scopes needed to handle the task.
Q: What happens if a user revokes access?
A: Arcade.dev will return appropriate errors that you can handle gracefully in your bot logic.
Resources
Ready to build more authenticated AI tools? Sign up for Arcade.dev